mirror of
https://github.com/Sarsoo/csbindgen.git
synced 2024-12-22 14:36:26 +00:00
wip, libphysx
This commit is contained in:
parent
1bd4f42ba2
commit
b39702c95c
1
csbindgen-tests/Cargo.toml
vendored
1
csbindgen-tests/Cargo.toml
vendored
@ -14,6 +14,7 @@ path = "src/lib.rs"
|
||||
|
||||
[dependencies]
|
||||
csbindgen = { path = "../csbindgen" }
|
||||
physx-sys = "0.11.0"
|
||||
|
||||
[build-dependencies]
|
||||
cc = "1.0.79"
|
||||
|
9
csbindgen-tests/build.rs
vendored
9
csbindgen-tests/build.rs
vendored
@ -125,5 +125,14 @@ fn main() -> Result<(), Box<dyn Error>> {
|
||||
.csharp_dll_name("libpng16")
|
||||
.generate_csharp_file("../dotnet-sandbox/libpng16_csbindgen.cs")?;
|
||||
|
||||
|
||||
csbindgen::Builder::new()
|
||||
.input_bindgen_file("src/physx/physx_generated.rs")
|
||||
.input_bindgen_file("src/physx/x86_64-pc-windows-msvc/structgen.rs")
|
||||
.csharp_namespace("Physx")
|
||||
.csharp_class_name("LibPhysxd")
|
||||
.csharp_dll_name("libphysx")
|
||||
.generate_csharp_file("../dotnet-sandbox/libphysx_csbindgen.cs")?;
|
||||
|
||||
Ok(())
|
||||
}
|
||||
|
34
csbindgen-tests/src/lib.rs
vendored
34
csbindgen-tests/src/lib.rs
vendored
@ -1,8 +1,10 @@
|
||||
use std::{
|
||||
collections::HashSet,
|
||||
ffi::{c_char, c_long, c_ulong, c_void, CString},
|
||||
ffi::{c_char, c_long, c_ulong, c_void, CString}, ptr::null_mut,
|
||||
};
|
||||
|
||||
use physx_sys::*;
|
||||
|
||||
#[allow(dead_code)]
|
||||
#[allow(non_snake_case)]
|
||||
#[allow(non_camel_case_types)]
|
||||
@ -546,3 +548,33 @@ pub struct CallbackTable {
|
||||
pub foo: extern "C" fn(),
|
||||
pub foobar: extern "C" fn(i: i32) -> i32,
|
||||
}
|
||||
|
||||
|
||||
fn run_physix(){
|
||||
unsafe {
|
||||
let foundation = physx_create_foundation();
|
||||
let physics = physx_create_physics(foundation);
|
||||
|
||||
let mut scene_desc = PxSceneDesc_new(PxPhysics_getTolerancesScale(physics));
|
||||
scene_desc.gravity = PxVec3 {
|
||||
x: 0.0,
|
||||
y: -9.81,
|
||||
z: 0.0,
|
||||
};
|
||||
|
||||
|
||||
|
||||
let dispatcher = phys_PxDefaultCpuDispatcherCreate(
|
||||
1,
|
||||
null_mut(),
|
||||
PxDefaultCpuDispatcherWaitForWorkMode::WaitForWork,
|
||||
0,
|
||||
);
|
||||
scene_desc.cpuDispatcher = dispatcher.cast();
|
||||
scene_desc.filterShader = get_default_simulation_filter_shader();
|
||||
|
||||
let scene = PxPhysics_createScene_mut(physics, &scene_desc);
|
||||
|
||||
// Your physics simulation goes here
|
||||
}
|
||||
}
|
408
csbindgen-tests/src/physx/lib.rs
vendored
Normal file
408
csbindgen-tests/src/physx/lib.rs
vendored
Normal file
@ -0,0 +1,408 @@
|
||||
//! # 🎳 physx-sys
|
||||
//!
|
||||
//! 
|
||||
//! [](https://crates.io/crates/physx-sys)
|
||||
//! [](https://docs.rs/physx-sys)
|
||||
//! [](../CODE_OF_CONDUCT.md)
|
||||
//! [](http://embark.games)
|
||||
//!
|
||||
//! Unsafe automatically-generated Rust bindings for [NVIDIA PhysX 4.1](https://github.com/NVIDIAGameWorks/PhysX) C++ API.
|
||||
//!
|
||||
//! Please also see the [repository](https://github.com/EmbarkStudios/physx-rs) containing a work-in-progress safe wrapper.
|
||||
//!
|
||||
//! ## Presentation
|
||||
//!
|
||||
//! [Tomasz Stachowiak](https://github.com/h3r2tic) did a presentation at the Stockholm Rust Meetup on October 2019
|
||||
//! about this project that goes through the tecnical details of how C++ to Rust bindings of `physx-sys` works:
|
||||
//!
|
||||
//! [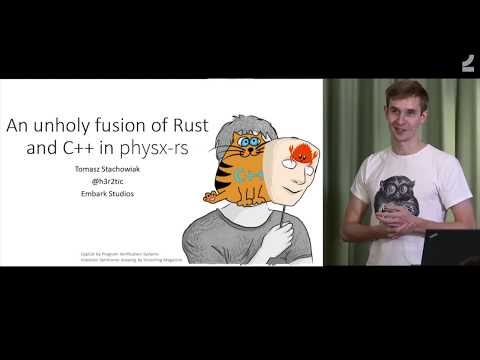](http://www.youtube.com/watch?v=RxtXGeDHu0w "An unholy fusion of
|
||||
//! Rust and C++ in physx-rs (Stockholm Rust Meetup, October 2019)")
|
||||
//!
|
||||
//!
|
||||
//! ## Basic usage
|
||||
//!
|
||||
//! ```Rust
|
||||
//! unsafe {
|
||||
//! let foundation = physx_create_foundation();
|
||||
//! let physics = physx_create_physics(foundation);
|
||||
//!
|
||||
//! let mut scene_desc = PxSceneDesc_new(PxPhysics_getTolerancesScale(physics));
|
||||
//! scene_desc.gravity = PxVec3 {
|
||||
//! x: 0.0,
|
||||
//! y: -9.81,
|
||||
//! z: 0.0,
|
||||
//! };
|
||||
//!
|
||||
//! let dispatcher = PxDefaultCpuDispatcherCreate(2, null_mut());
|
||||
//!
|
||||
//! scene_desc.cpuDispatcher = dispatcher as *mut PxCpuDispatcher;
|
||||
//! scene_desc.filterShader = Some(PxDefaultSimulationFilterShader);
|
||||
//!
|
||||
//! let scene = PxPhysics_createScene_mut(physics, &scene_desc);
|
||||
//!
|
||||
//! // Your physics simulation goes here
|
||||
//! }
|
||||
//! ```
|
||||
//!
|
||||
//! ## Examples
|
||||
//!
|
||||
//! ### [Ball](examples/ball.rs)
|
||||
//!
|
||||
//! A simple example to showcase how to use physx-sys. It can be run with `cargo run --examples ball`.
|
||||
//!
|
||||
//! ```
|
||||
//! o
|
||||
//!
|
||||
//! o
|
||||
//! o
|
||||
//!
|
||||
//! o
|
||||
//! ooooooooo
|
||||
//! o oo oo
|
||||
//! o o
|
||||
//! o o o
|
||||
//! o oo
|
||||
//! o o o
|
||||
//! o ooooooo
|
||||
//! o o oo oo
|
||||
//! o o o oo oo
|
||||
//! o o o o ooooooooo
|
||||
//! o o o oo oooooooooo oo
|
||||
//!
|
||||
//! ```
|
||||
//!
|
||||
//! ## How it works
|
||||
//!
|
||||
//! The binding is generated using a custom C++ app written against clang's
|
||||
//! [libtooling](https://clang.llvm.org/docs/LibTooling.html). It queries the compiler's abstract syntax tree, and maps
|
||||
//! the C++ PhysX functions and types to Rust using heuristics chosen specifically for this SDK. It is not a general
|
||||
//! C++ <-> Rust binding generator, and using it on other projects *will* likely crash and burn.
|
||||
//!
|
||||
//! Since C++ does not have a standardized and stable ABI, it's generally not safe to call it from Rust code; since
|
||||
//! PhysX exposes a C++ interface, we can't use it directly. That's why `physx-sys` generates both a Rust interface as
|
||||
//! well as a plain C wrapper. The C code is compiled into a static library at build time, and Rust then talks to C.
|
||||
//!
|
||||
//! In order to minimize the amount of work required to marshall data between the C wrapper and the original C++ API, we
|
||||
//! generate a **bespoke C wrapper for each build target**. The wrapper is based on metadata about structure layout
|
||||
//! extracted directly from compiling and running a tiny program against the PhysX SDK using the specific C++ compiler
|
||||
//! used in the build process.
|
||||
//!
|
||||
//! The build process comprises a few steps:
|
||||
//!
|
||||
//! 1. The `pxbind` utility uses `clang` to extract metadata about PhysX functions and types, and generates partial
|
||||
//! Rust and C bindings as `physx_generated.hpp` and `physx_generated.rs`. Those contain all function definitions, and
|
||||
//! a small subset of types. It also generates a C++ utility called `structgen` by emitting `structgen.cpp`.
|
||||
//! 2. `structgen` is compiled against the PhysX SDK, and generates all the remaining type wrappers. For each struct, it
|
||||
//! queries the size and offset of its members, and generates `structgen_out.hpp` and `structgen_out.rs`. The types are
|
||||
//! "plain old data" structs which will perfectly match the memory layout of the C++ types.
|
||||
//! 3. All the generated C types are compiled together to form `physx_api`, a static library for Rust to link with.
|
||||
//! 4. The Rust wrapper is compiled, and linked with PhysX and the C wrapper.
|
||||
//!
|
||||
//! Steps *2..4* are performed completely automatically from within `build.rs`, while step *1* is only necessary when
|
||||
//! upgrading the PhysX SDK or modifying the generator. As such, building and running `pxbind` is a manual task, and is
|
||||
//! currently only supported on \*nix systems.
|
||||
//!
|
||||
//! ## License
|
||||
//!
|
||||
//! Licensed under either of
|
||||
//!
|
||||
//! * Apache License, Version 2.0, ([LICENSE-APACHE](LICENSE-APACHE) or http://www.apache.org/licenses/LICENSE-2.0)
|
||||
//! * MIT license ([LICENSE-MIT](LICENSE-MIT) or http://opensource.org/licenses/MIT)
|
||||
//!
|
||||
//! at your option.
|
||||
//!
|
||||
//! Note that the [PhysX C++ SDK](https://github.com/NVIDIAGameWorks/PhysX) has it's
|
||||
//! [own BSD 3 license](https://gameworksdocs.nvidia.com/PhysX/4.1/documentation/physxguide/Manual/License.html) and
|
||||
//! depends on [additional C++ third party libraries](https://github.com/NVIDIAGameWorks/PhysX/tree/4.1/externals).
|
||||
//!
|
||||
//! ### Contribution
|
||||
//!
|
||||
//! Unless you explicitly state otherwise, any contribution intentionally
|
||||
//! submitted for inclusion in the work by you, as defined in the Apache-2.0
|
||||
//! license, shall be dual licensed as above, without any additional terms or
|
||||
//! conditions.
|
||||
|
||||
// crate-specific exceptions:
|
||||
#![allow(
|
||||
unsafe_code,
|
||||
non_upper_case_globals,
|
||||
non_camel_case_types,
|
||||
non_snake_case,
|
||||
clippy::doc_markdown, // TODO: fixup comments and docs (though annoyingly complains about "PhysX")
|
||||
clippy::unreadable_literal,
|
||||
clippy::unused_unit,
|
||||
clippy::upper_case_acronyms
|
||||
)]
|
||||
|
||||
#[cfg(feature = "structgen")]
|
||||
include!(concat!(env!("OUT_DIR"), "/structgen_out.rs"));
|
||||
|
||||
#[cfg(all(
|
||||
not(feature = "structgen"),
|
||||
target_os = "linux",
|
||||
target_arch = "x86_64",
|
||||
))]
|
||||
include!("generated/unix/structgen.rs");
|
||||
|
||||
#[cfg(all(
|
||||
not(feature = "structgen"),
|
||||
target_os = "linux",
|
||||
target_arch = "aarch64",
|
||||
))]
|
||||
include!("generated/unix/structgen.rs");
|
||||
|
||||
#[cfg(all(
|
||||
not(feature = "structgen"),
|
||||
target_os = "android",
|
||||
target_arch = "aarch64",
|
||||
))]
|
||||
include!("generated/unix/structgen.rs");
|
||||
|
||||
#[cfg(all(
|
||||
not(feature = "structgen"),
|
||||
target_os = "macos",
|
||||
target_arch = "x86_64",
|
||||
))]
|
||||
include!("generated/unix/structgen.rs");
|
||||
|
||||
#[cfg(all(
|
||||
not(feature = "structgen"),
|
||||
target_os = "macos",
|
||||
target_arch = "aarch64",
|
||||
))]
|
||||
include!("generated/unix/structgen.rs");
|
||||
|
||||
#[cfg(all(
|
||||
not(feature = "structgen"),
|
||||
target_os = "windows",
|
||||
target_arch = "x86_64",
|
||||
target_env = "msvc",
|
||||
))]
|
||||
include!("generated/x86_64-pc-windows-msvc/structgen.rs");
|
||||
|
||||
include!("physx_generated.rs");
|
||||
|
||||
use std::ffi::c_void;
|
||||
|
||||
pub const fn version(major: u32, minor: u32, patch: u32) -> u32 {
|
||||
(major << 24) + (minor << 16) + (patch << 8)
|
||||
}
|
||||
|
||||
pub type CollisionCallback =
|
||||
unsafe extern "C" fn(*mut c_void, *const PxContactPairHeader, *const PxContactPair, u32);
|
||||
|
||||
pub type TriggerCallback = unsafe extern "C" fn(*mut c_void, *const PxTriggerPair, u32);
|
||||
|
||||
pub type ConstraintBreakCallback = unsafe extern "C" fn(*mut c_void, *const PxConstraintInfo, u32);
|
||||
|
||||
pub type WakeSleepCallback = unsafe extern "C" fn(*mut c_void, *const *const PxActor, u32, bool);
|
||||
|
||||
pub type AdvanceCallback =
|
||||
unsafe extern "C" fn(*mut c_void, *const *const PxRigidBody, *const PxTransform, u32);
|
||||
|
||||
// Function pointers in Rust are normally not nullable (which is why they don't require unsafe to call)
|
||||
// but we need them to be, so we simply wrap them in Option<>. An Option<funcptr> is luckily represented
|
||||
// by the compiler as a simple pointer with null representing None, so this is compatible with the C struct.
|
||||
#[repr(C)]
|
||||
pub struct SimulationEventCallbackInfo {
|
||||
// Callback for collision events.
|
||||
pub collision_callback: Option<CollisionCallback>,
|
||||
pub collision_user_data: *mut c_void,
|
||||
// Callback for trigger shape events (an object entered or left a trigger shape).
|
||||
pub trigger_callback: Option<TriggerCallback>,
|
||||
pub trigger_user_data: *mut c_void,
|
||||
// Callback for when a constraint breaks (such as a joint with a force limit)
|
||||
pub constraint_break_callback: Option<ConstraintBreakCallback>,
|
||||
pub constraint_break_user_data: *mut c_void,
|
||||
// Callback for when an object falls asleep or is awoken.
|
||||
pub wake_sleep_callback: Option<WakeSleepCallback>,
|
||||
pub wake_sleep_user_data: *mut c_void,
|
||||
// Callback to get the next pose early for objects (if flagged with eENABLE_POSE_INTEGRATION_PREVIEW).
|
||||
pub advance_callback: Option<AdvanceCallback>,
|
||||
pub advance_user_data: *mut c_void,
|
||||
}
|
||||
|
||||
impl Default for SimulationEventCallbackInfo {
|
||||
fn default() -> Self {
|
||||
Self {
|
||||
collision_callback: None,
|
||||
collision_user_data: std::ptr::null_mut(),
|
||||
trigger_callback: None,
|
||||
trigger_user_data: std::ptr::null_mut(),
|
||||
constraint_break_callback: None,
|
||||
constraint_break_user_data: std::ptr::null_mut(),
|
||||
wake_sleep_callback: None,
|
||||
wake_sleep_user_data: std::ptr::null_mut(),
|
||||
advance_callback: None,
|
||||
advance_user_data: std::ptr::null_mut(),
|
||||
}
|
||||
}
|
||||
}
|
||||
|
||||
/// return 0 = `PxQueryHitType::eNONE`
|
||||
/// return 1 = `PxQueryHitType::eTOUCH`
|
||||
/// return 2 = `PxQueryHitType::eBLOCK`
|
||||
pub type RaycastHitCallback = unsafe extern "C" fn(
|
||||
*const PxRigidActor,
|
||||
*const PxFilterData,
|
||||
*const PxShape,
|
||||
hit_flags: u32,
|
||||
*const c_void,
|
||||
) -> PxQueryHitType;
|
||||
|
||||
#[repr(C)]
|
||||
pub struct FilterShaderCallbackInfo {
|
||||
pub attributes0: u32,
|
||||
pub attributes1: u32,
|
||||
pub filterData0: PxFilterData,
|
||||
pub filterData1: PxFilterData,
|
||||
pub pairFlags: *mut PxPairFlags,
|
||||
pub constantBlock: *const std::ffi::c_void,
|
||||
pub constantBlockSize: u32,
|
||||
}
|
||||
|
||||
pub type SimulationFilterShader =
|
||||
unsafe extern "C" fn(*mut FilterShaderCallbackInfo) -> PxFilterFlags;
|
||||
|
||||
pub type RaycastProcessTouchesCallback =
|
||||
unsafe extern "C" fn(*const PxRaycastHit, u32, *mut c_void) -> bool;
|
||||
pub type SweepProcessTouchesCallback =
|
||||
unsafe extern "C" fn(*const PxSweepHit, u32, *mut c_void) -> bool;
|
||||
pub type OverlapProcessTouchesCallback =
|
||||
unsafe extern "C" fn(*const PxOverlapHit, u32, *mut c_void) -> bool;
|
||||
|
||||
pub type FinalizeQueryCallback = unsafe extern "C" fn(*mut c_void);
|
||||
|
||||
pub type AllocCallback =
|
||||
unsafe extern "C" fn(u64, *const c_void, *const c_void, u32, *const c_void) -> *mut c_void;
|
||||
|
||||
pub type DeallocCallback = unsafe extern "C" fn(*const c_void, *const c_void);
|
||||
|
||||
pub type ZoneStartCallback =
|
||||
unsafe extern "C" fn(*const i8, bool, u64, *const c_void) -> *mut c_void;
|
||||
|
||||
pub type ZoneEndCallback = unsafe extern "C" fn(*const c_void, *const i8, bool, u64, *const c_void);
|
||||
|
||||
pub type ErrorCallback =
|
||||
unsafe extern "C" fn(PxErrorCode, *const i8, *const i8, u32, *const c_void);
|
||||
|
||||
pub type AssertHandler = unsafe extern "C" fn(*const i8, *const i8, u32, *mut bool, *const c_void);
|
||||
|
||||
extern "C" {
|
||||
pub fn physx_create_foundation() -> *mut PxFoundation;
|
||||
pub fn physx_create_foundation_with_alloc(
|
||||
allocator: *mut PxDefaultAllocator,
|
||||
) -> *mut PxFoundation;
|
||||
pub fn physx_create_physics(foundation: *mut PxFoundation) -> *mut PxPhysics;
|
||||
|
||||
pub fn get_default_allocator() -> *mut PxDefaultAllocator;
|
||||
pub fn get_default_error_callback() -> *mut PxDefaultErrorCallback;
|
||||
|
||||
/// Destroy the returned callback object using PxQueryFilterCallback_delete.
|
||||
pub fn create_raycast_filter_callback(
|
||||
actor_to_ignore: *const PxRigidActor,
|
||||
) -> *mut PxQueryFilterCallback;
|
||||
|
||||
/// Destroy the returned callback object using PxQueryFilterCallback_delete.
|
||||
pub fn create_raycast_filter_callback_func(
|
||||
callback: RaycastHitCallback,
|
||||
userdata: *mut c_void,
|
||||
) -> *mut PxQueryFilterCallback;
|
||||
|
||||
pub fn create_raycast_buffer() -> *mut PxRaycastCallback;
|
||||
pub fn create_sweep_buffer() -> *mut PxSweepCallback;
|
||||
pub fn create_overlap_buffer() -> *mut PxOverlapCallback;
|
||||
|
||||
pub fn create_raycast_callback(
|
||||
process_touches_callback: RaycastProcessTouchesCallback,
|
||||
finalize_query_callback: FinalizeQueryCallback,
|
||||
touches_buffer: *mut PxRaycastHit,
|
||||
num_touches: u32,
|
||||
userdata: *mut c_void,
|
||||
) -> *mut PxRaycastCallback;
|
||||
pub fn create_sweep_callback(
|
||||
process_touches_callback: SweepProcessTouchesCallback,
|
||||
finalize_query_callback: FinalizeQueryCallback,
|
||||
touches_buffer: *mut PxSweepHit,
|
||||
num_touches: u32,
|
||||
userdata: *mut c_void,
|
||||
) -> *mut PxSweepCallback;
|
||||
pub fn create_overlap_callback(
|
||||
process_touches_callback: OverlapProcessTouchesCallback,
|
||||
finalize_query_callback: FinalizeQueryCallback,
|
||||
touches_buffer: *mut PxOverlapHit,
|
||||
num_touches: u32,
|
||||
userdata: *mut c_void,
|
||||
) -> *mut PxOverlapCallback;
|
||||
|
||||
pub fn delete_raycast_callback(callback: *mut PxRaycastCallback);
|
||||
pub fn delete_sweep_callback(callback: *mut PxSweepCallback);
|
||||
pub fn delete_overlap_callback(callback: *mut PxOverlapCallback);
|
||||
|
||||
pub fn create_alloc_callback(
|
||||
alloc_callback: AllocCallback,
|
||||
dealloc_callback: DeallocCallback,
|
||||
userdata: *mut c_void,
|
||||
) -> *mut PxAllocatorCallback;
|
||||
|
||||
pub fn create_profiler_callback(
|
||||
zone_start_callback: ZoneStartCallback,
|
||||
zone_end_callback: ZoneEndCallback,
|
||||
userdata: *mut c_void,
|
||||
) -> *mut PxProfilerCallback;
|
||||
|
||||
pub fn get_alloc_callback_user_data(alloc_callback: *mut PxAllocatorCallback) -> *mut c_void;
|
||||
|
||||
pub fn create_error_callback(
|
||||
error_callback: ErrorCallback,
|
||||
userdata: *mut c_void,
|
||||
) -> *mut PxErrorCallback;
|
||||
|
||||
pub fn create_assert_handler(
|
||||
error_callback: AssertHandler,
|
||||
userdata: *mut c_void,
|
||||
) -> *mut PxAssertHandler;
|
||||
|
||||
pub fn get_default_simulation_filter_shader() -> *mut c_void;
|
||||
|
||||
/// Create a C++ proxy callback which will forward contact events to `Callback`.
|
||||
/// The returned pointer must be freed by calling `destroy_contact_callback` when done using.
|
||||
#[deprecated]
|
||||
pub fn create_contact_callback(
|
||||
callback: CollisionCallback,
|
||||
userdata: *mut c_void,
|
||||
) -> *mut PxSimulationEventCallback;
|
||||
/// Deallocates the PxSimulationEventCallback that has previously been created
|
||||
#[deprecated()]
|
||||
pub fn destroy_contact_callback(callback: *mut PxSimulationEventCallback);
|
||||
|
||||
/// New interface to handle simulation events, replacing create_contact_callback.
|
||||
pub fn create_simulation_event_callbacks(
|
||||
callbacks: *const SimulationEventCallbackInfo,
|
||||
) -> *mut PxSimulationEventCallback;
|
||||
|
||||
pub fn get_simulation_event_info(
|
||||
callback: *mut PxSimulationEventCallback,
|
||||
) -> *mut SimulationEventCallbackInfo;
|
||||
|
||||
pub fn destroy_simulation_event_callbacks(callback: *mut PxSimulationEventCallback);
|
||||
|
||||
/// Override the default filter shader in the scene with a custom function.
|
||||
/// If call_default_filter_shader_first is set to true, this will first call the
|
||||
/// built-in PhysX filter (that matches Physx 2.8 behavior) before your callback.
|
||||
pub fn enable_custom_filter_shader(
|
||||
scene_desc: *mut PxSceneDesc,
|
||||
shader: SimulationFilterShader,
|
||||
call_default_filter_shader_first: u32,
|
||||
);
|
||||
|
||||
#[doc(hidden)]
|
||||
/// Should only be used in testing etc! This isn't generated as we don't generate op functions.
|
||||
pub fn PxAssertHandler_opCall_mut(
|
||||
self_: *mut PxAssertHandler,
|
||||
expr: *const i8,
|
||||
file: *const i8,
|
||||
line: i32,
|
||||
ignore: *mut bool,
|
||||
) -> ();
|
||||
}
|
14250
csbindgen-tests/src/physx/physx_generated.rs
vendored
Normal file
14250
csbindgen-tests/src/physx/physx_generated.rs
vendored
Normal file
File diff suppressed because it is too large
Load Diff
2815
csbindgen-tests/src/physx/unix/structgen.rs
vendored
Normal file
2815
csbindgen-tests/src/physx/unix/structgen.rs
vendored
Normal file
File diff suppressed because it is too large
Load Diff
2822
csbindgen-tests/src/physx/x86_64-pc-windows-msvc/structgen.rs
vendored
Normal file
2822
csbindgen-tests/src/physx/x86_64-pc-windows-msvc/structgen.rs
vendored
Normal file
File diff suppressed because it is too large
Load Diff
@ -12,7 +12,7 @@ pub struct Builder {
|
||||
}
|
||||
|
||||
pub struct BindgenOptions {
|
||||
pub input_bindgen_file: String,
|
||||
pub input_bindgen_files: Vec<String>,
|
||||
pub input_extern_files: Vec<String>,
|
||||
pub method_filter: fn(method_name: String) -> bool,
|
||||
pub rust_method_type_path: String,
|
||||
@ -33,7 +33,7 @@ impl Default for Builder {
|
||||
fn default() -> Self {
|
||||
Self {
|
||||
options: BindgenOptions {
|
||||
input_bindgen_file: "".to_string(),
|
||||
input_bindgen_files: vec![],
|
||||
input_extern_files: vec![],
|
||||
method_filter: |x| !x.starts_with('_'),
|
||||
rust_method_type_path: "".to_string(),
|
||||
@ -60,7 +60,9 @@ impl Builder {
|
||||
|
||||
/// Change an input .rs file(such as generated from bindgen) to generate binding.
|
||||
pub fn input_bindgen_file<T: Into<String>>(mut self, input_bindgen_file: T) -> Builder {
|
||||
self.options.input_bindgen_file = input_bindgen_file.into();
|
||||
self.options
|
||||
.input_bindgen_files
|
||||
.push(input_bindgen_file.into());
|
||||
self
|
||||
}
|
||||
|
||||
@ -165,7 +167,7 @@ impl Builder {
|
||||
&self,
|
||||
csharp_output_path: P,
|
||||
) -> Result<(), Box<dyn Error>> {
|
||||
if !self.options.input_bindgen_file.is_empty() {
|
||||
if !self.options.input_bindgen_files.is_empty() {
|
||||
let (_, csharp) = generate(GenerateKind::InputBindgen, &self.options)?;
|
||||
|
||||
let mut csharp_file = make_file(csharp_output_path.as_ref())?;
|
||||
@ -189,7 +191,7 @@ impl Builder {
|
||||
rust_output_path: P,
|
||||
csharp_output_path: P,
|
||||
) -> Result<(), Box<dyn Error>> {
|
||||
if !self.options.input_bindgen_file.is_empty() {
|
||||
if !self.options.input_bindgen_files.is_empty() {
|
||||
let (rust, csharp) = generate(GenerateKind::InputBindgen, &self.options)?;
|
||||
|
||||
if let Some(rust) = rust {
|
||||
|
@ -25,9 +25,8 @@ pub(crate) fn generate(
|
||||
generate_kind: GenerateKind,
|
||||
options: &BindgenOptions,
|
||||
) -> Result<(Option<String>, String), Box<dyn Error>> {
|
||||
let temp_input = [options.input_bindgen_file.clone()];
|
||||
let (paths, generate_rust) = match generate_kind {
|
||||
GenerateKind::InputBindgen => (temp_input.as_slice(), true),
|
||||
GenerateKind::InputBindgen => (options.input_bindgen_files.as_slice(), true),
|
||||
GenerateKind::InputExtern => (options.input_extern_files.as_slice(), false),
|
||||
};
|
||||
|
||||
|
10738
dotnet-sandbox/libphysx_csbindgen.cs
vendored
Normal file
10738
dotnet-sandbox/libphysx_csbindgen.cs
vendored
Normal file
File diff suppressed because it is too large
Load Diff
@ -43,7 +43,7 @@ MonoBehaviour:
|
||||
width: 1953
|
||||
height: 1546
|
||||
m_ShowMode: 4
|
||||
m_Title: Game
|
||||
m_Title: Console
|
||||
m_RootView: {fileID: 11}
|
||||
m_MinSize: {x: 875, y: 300}
|
||||
m_MaxSize: {x: 10000, y: 10000}
|
||||
@ -97,7 +97,7 @@ MonoBehaviour:
|
||||
m_MinSize: {x: 640, y: 601}
|
||||
m_MaxSize: {x: 4000, y: 4021}
|
||||
vertical: 0
|
||||
controlID: 2323
|
||||
controlID: 123
|
||||
--- !u!114 &5
|
||||
MonoBehaviour:
|
||||
m_ObjectHideFlags: 52
|
||||
@ -148,7 +148,7 @@ MonoBehaviour:
|
||||
m_MinSize: {x: 200, y: 100}
|
||||
m_MaxSize: {x: 16192, y: 8096}
|
||||
vertical: 0
|
||||
controlID: 24
|
||||
controlID: 231
|
||||
--- !u!114 &7
|
||||
MonoBehaviour:
|
||||
m_ObjectHideFlags: 52
|
||||
@ -173,7 +173,7 @@ MonoBehaviour:
|
||||
m_MinSize: {x: 300, y: 200}
|
||||
m_MaxSize: {x: 24288, y: 16192}
|
||||
vertical: 0
|
||||
controlID: 17
|
||||
controlID: 108
|
||||
--- !u!114 &8
|
||||
MonoBehaviour:
|
||||
m_ObjectHideFlags: 52
|
||||
@ -193,8 +193,8 @@ MonoBehaviour:
|
||||
y: 0
|
||||
width: 456
|
||||
height: 1496
|
||||
m_MinSize: {x: 276, y: 71}
|
||||
m_MaxSize: {x: 4001, y: 4021}
|
||||
m_MinSize: {x: 275, y: 50}
|
||||
m_MaxSize: {x: 4000, y: 4000}
|
||||
m_ActualView: {fileID: 20}
|
||||
m_Panes:
|
||||
- {fileID: 20}
|
||||
@ -236,7 +236,7 @@ MonoBehaviour:
|
||||
m_Enabled: 1
|
||||
m_EditorHideFlags: 1
|
||||
m_Script: {fileID: 12006, guid: 0000000000000000e000000000000000, type: 0}
|
||||
m_Name: ProjectSettingsWindow
|
||||
m_Name: ConsoleWindow
|
||||
m_EditorClassIdentifier:
|
||||
m_Children: []
|
||||
m_Position:
|
||||
@ -245,14 +245,14 @@ MonoBehaviour:
|
||||
y: 0
|
||||
width: 997
|
||||
height: 608
|
||||
m_MinSize: {x: 311, y: 221}
|
||||
m_MinSize: {x: 101, y: 121}
|
||||
m_MaxSize: {x: 4001, y: 4021}
|
||||
m_ActualView: {fileID: 18}
|
||||
m_ActualView: {fileID: 24}
|
||||
m_Panes:
|
||||
- {fileID: 24}
|
||||
- {fileID: 18}
|
||||
m_Selected: 1
|
||||
m_LastSelected: 0
|
||||
m_Selected: 0
|
||||
m_LastSelected: 1
|
||||
--- !u!114 &11
|
||||
MonoBehaviour:
|
||||
m_ObjectHideFlags: 52
|
||||
@ -348,7 +348,7 @@ MonoBehaviour:
|
||||
m_MinSize: {x: 200, y: 200}
|
||||
m_MaxSize: {x: 16192, y: 16192}
|
||||
vertical: 1
|
||||
controlID: 123
|
||||
controlID: 109
|
||||
--- !u!114 &15
|
||||
MonoBehaviour:
|
||||
m_ObjectHideFlags: 52
|
||||
@ -373,7 +373,7 @@ MonoBehaviour:
|
||||
m_MinSize: {x: 200, y: 100}
|
||||
m_MaxSize: {x: 16192, y: 8096}
|
||||
vertical: 0
|
||||
controlID: 124
|
||||
controlID: 110
|
||||
--- !u!114 &16
|
||||
MonoBehaviour:
|
||||
m_ObjectHideFlags: 52
|
||||
@ -560,9 +560,9 @@ MonoBehaviour:
|
||||
m_IsLocked: 0
|
||||
m_FolderTreeState:
|
||||
scrollPos: {x: 0, y: 0}
|
||||
m_SelectedIDs: b65f0000
|
||||
m_LastClickedID: 24502
|
||||
m_ExpandedIDs: 00000000b65f000000ca9a3bffffff7f
|
||||
m_SelectedIDs: de5f0000
|
||||
m_LastClickedID: 24542
|
||||
m_ExpandedIDs: 00000000de5f000000ca9a3bffffff7f
|
||||
m_RenameOverlay:
|
||||
m_UserAcceptedRename: 0
|
||||
m_Name:
|
||||
@ -590,7 +590,7 @@ MonoBehaviour:
|
||||
scrollPos: {x: 0, y: 0}
|
||||
m_SelectedIDs:
|
||||
m_LastClickedID: 0
|
||||
m_ExpandedIDs: 00000000b65f0000
|
||||
m_ExpandedIDs: 00000000de5f0000
|
||||
m_RenameOverlay:
|
||||
m_UserAcceptedRename: 0
|
||||
m_Name:
|
||||
@ -617,7 +617,7 @@ MonoBehaviour:
|
||||
m_ListAreaState:
|
||||
m_SelectedInstanceIDs:
|
||||
m_LastClickedInstanceID: 0
|
||||
m_HadKeyboardFocusLastEvent: 1
|
||||
m_HadKeyboardFocusLastEvent: 0
|
||||
m_ExpandedInstanceIDs: c6230000
|
||||
m_RenameOverlay:
|
||||
m_UserAcceptedRename: 0
|
||||
@ -720,21 +720,21 @@ MonoBehaviour:
|
||||
scrollPos: {x: 0, y: 0}
|
||||
m_SelectedIDs:
|
||||
m_LastClickedID: 0
|
||||
m_ExpandedIDs: 6ccbffffb6cbffff16cdffff84cfffffcecfffff2ed1ffff2cd5ffff76d5ffffd6d6ffff2ad9ffff74d9ffffd4daffff1eddffff68ddffffc8deffff24e1ffff6ee1ffffcee2ffff74e6ffffbee6ffff1ee8ffff26eaffff70eaffffd0ebfffffcf3ffff8af4ffffccf4ffff3af6ffff22fbffff78780000
|
||||
m_ExpandedIDs: 18d4ffff60d4ffffc0d5ffff12d8ffff5ad8ffffbad9ffff04dcffff4cdcffffacddfffff6dfffff3ee0ffff58e0ffff00e1ffff48e1ffffa8e2ffff90e6ffffd8e6ffff38e8ffff5cebffffa4ebffff04edffff2afbffff
|
||||
m_RenameOverlay:
|
||||
m_UserAcceptedRename: 0
|
||||
m_Name: Text (Legacy)
|
||||
m_OriginalName: Text (Legacy)
|
||||
m_Name:
|
||||
m_OriginalName:
|
||||
m_EditFieldRect:
|
||||
serializedVersion: 2
|
||||
x: 0
|
||||
y: 0
|
||||
width: 0
|
||||
height: 0
|
||||
m_UserData: -3120
|
||||
m_UserData: 0
|
||||
m_IsWaitingForDelay: 0
|
||||
m_IsRenaming: 0
|
||||
m_OriginalEventType: 4
|
||||
m_OriginalEventType: 11
|
||||
m_IsRenamingFilename: 0
|
||||
m_ClientGUIView: {fileID: 9}
|
||||
m_SearchString:
|
||||
@ -991,9 +991,9 @@ MonoBehaviour:
|
||||
m_PlayAudio: 0
|
||||
m_AudioPlay: 0
|
||||
m_Position:
|
||||
m_Target: {x: 639.1462, y: 631.1552, z: -5.5470376}
|
||||
m_Target: {x: 499.8913, y: 522.92053, z: 0}
|
||||
speed: 2
|
||||
m_Value: {x: 639.1462, y: 631.1552, z: -5.5470376}
|
||||
m_Value: {x: 499.8913, y: 522.92053, z: 0}
|
||||
m_RenderMode: 0
|
||||
m_CameraMode:
|
||||
drawMode: 0
|
||||
@ -1044,9 +1044,9 @@ MonoBehaviour:
|
||||
speed: 2
|
||||
m_Value: {x: 0, y: 0, z: 0, w: 1}
|
||||
m_Size:
|
||||
m_Target: 636.09985
|
||||
m_Target: 494.99554
|
||||
speed: 2
|
||||
m_Value: 636.09985
|
||||
m_Value: 494.99554
|
||||
m_Ortho:
|
||||
m_Target: 1
|
||||
speed: 2
|
||||
|
Loading…
Reference in New Issue
Block a user